Event Framework is an Open Source CloudEvents Framework for .NET applications. You include it in your .NET Core 3.1/.NET 6 app and it helps you to create, receive, send and to handle CloudEvents. After reaching 1.0.0-alpha.0.100 (with the first alpha dating back to early 2020), Event Framework is now available in beta form, with the 1.0.0-beta.1.1 release.
How to get started
The easiest way to get started with Event Framework is to include the package Weikio.EventFramework.AspNetCore in your ASP.NET Core based application and then to register the required bits and pieces into service container:
services.AddEventFramework()
Main features
The main features of the Event Framework include:
1. Create CloudEvents using CloudEventCreator
2. Send & Receive CloudEvents using Channels and Event Sources
3. Build Event Flows
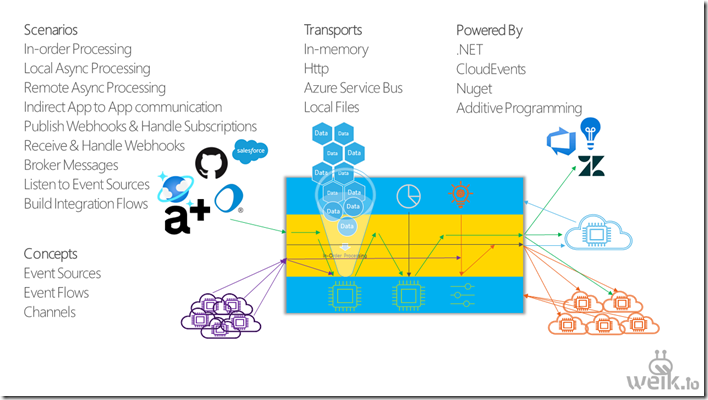
Here’s a short example of each of those:
Creating CloudEvents
Event Framework includes CloudEventCreator which can be used to transform .NET objects into CloudEvents. It can be customized and used through either a static CloudEventCreator.Create-method or using a CloudEventCreator instance.
var obj = new CustomerCreated(Guid.NewGuid(), "Test", "Customer");
// Object to event
var cloudEvent = CloudEventCreator.Create(obj);
// Object to event customization
var cloudEventCustomName = CloudEventCreator.Create(obj, eventTypeName: "custom-name");
For more examples, please see the following tests in the Github repo: https://github.com/weikio/EventFramework/tree/master/tests/unit/Weikio.EventFramework.UnitTests
Sending CloudEvents
Event Framework uses Channels when transporting and transforming events from a source to an endpoint. Channels have adapters, components and endpoints (+ interceptors) which are used to process an event. Channels can be build using a fluent builder or more manually.

Here's an example where a channel is created using the fluent builder with a single HTTP endpoint. Every object sent to this channel is transformed to CloudEvent and then delivered using HTTP:
var channel = await CloudEventsChannelBuilder.From("myHttpChannel")
.Http("https://webhook.site/3bdf5c39-065b-48f8-8356-511b284de874")
.Build(serviceProvider);
await channel.Send(new CustomerCreatedEvent() { Age = 50, Name = "Test User" });
In many situations in your applications you don’t send messages directly into the channel. Instead you inject ICloudEventPublisher into your controller/service and use it to publish events to a particular channel or to the default channel:
public IntegrationEndpointService(ICloudEventPublisher eventPublisher)
{
_eventPublisher = eventPublisher;
}
...
await _eventPublisher.Publish(new EndpointCreated()
{
Name = endpoint.Name,
Route = endpoint.Route,
ApiName = endpoint.ApiName,
ApiVersion = endpoint.ApiVersion,
EndpointId = result.Id.GetValueOrDefault(),
});
Receiving CloudEvents
Event Framework supports Event Sources. An event source can be used to receive events (for example: HTTP, Azure Service Bus) but an event source can also poll and watch changes happening in some other system (like local file system).
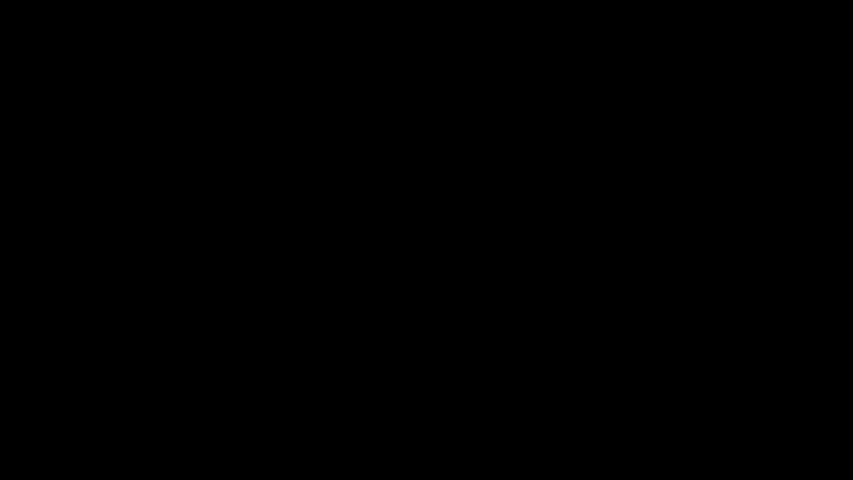
Here's an example where HTTP and Azure Service Bus are used to receive events in ASP.NET Core and then logged:
services.AddEventFramework()
.AddChannel(CloudEventsChannelBuilder.From("logChannel")
.Logger())
.AddHttpCloudEventSource("events")
.AddAzureServiceBusCloudEventSource(
"Endpoint=sb://sb.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=YDcvmuL4=",
"myqueue");
services.Configure<DefaultChannelOptions>(options => options.DefaultChannelName = "logChannel");
Building Event Flows
Event Sources and Channels can be combined into Event Flows. Event Flows also support branching and subflows.
Here's an example where an event source is used to track file changes in a local file system and then all the created files are reported using HTTP:
var flow = EventFlowBuilder.From<FileSystemEventSource>(options =>
{
options.Configuration = new FileSystemEventSourceConfiguration() { Folder = @"c:\\temp\\myfiles", Filter = "*.bin" };
})
.Filter(ev => ev.Type == "FileCreatedEvent" ? Filter.Continue : Filter.Skip)
.Http("https://webhook.site/3bdf5c39-065b-48f8-8356-511b284de874");
services.AddEventFramework()
.AddEventFlow(flow);
Coming Next
Current document is lacking and samples also need work. The hope is to be able to include as many components and event sources as possible and for these, we’re looking at maybe using Apache Camel to bootstrap things.
Project Home
Please visit the project home site at https://weik.io/eventframework for more details. Though for now, the details are quite thin.
Source code
Source code for Event Framework is available from https://github.com/weikio/EventFramework.